
Java 高级程序设计:作业 06
Java 高级程序设计:实验六 二进制 I/O
代码地址:Github
实验目的和要求
-
能操作处理文本I/O。
-
能使用二进制I/O类 FileInputStream和FileOutputStream。
-
能使用二进制I/O类 FilterInputStream和FilterOutputStream。
-
能使用二进制I/O类 DataInputStream和DataOutputStream。
-
能使用二进制I/O类 BufferedInputStream和BufferedOutputStream。
-
能使用对象I/O类实现序列化。
实验内容
1、(创建文本文件)编写程序,如果文件 高级程序设计.txt不存在,则创建该文件。如果存在,则向该文件追加新数据。使用文本I/O将100个随机生成的整数写入这个文件,整数间用空格隔开。
2、(存储Loan对象)编写程序清单如10-2所示,重写Loan类实现Serializable。编写程序创建5个Loan对象并利用ObjectOutputStream将它们存储在一个文件LoanInfo.dat文件中。
3、(从文件中恢复对象)利用前面一题存储的文件LoanInfo.dat文件,编写程序从上述文件中读取内容到Loan对象中,并计算贷款总额并打印到控制台上。
4、(字符频率)编写程序,提示用户输入一个ASCII文本文件名,然后显示文件中每个字符出现的频率。
实验结果
Answer01
import java.io.File;
import java.io.FileWriter;
import java.io.IOException;
import java.util.Random;
/**
* @author Owem
* @date 2022/10/25 15:39
* @description TODO
**/
public class CreateTextFile {
private static void addNumbers(File file) {
int number;
try (FileWriter fw = new FileWriter(file, true)) {
for (int i = 0; i < 100; i++) {
number = (int)(Math.random() * (100 + 1));
fw.write(number + " ");
}
fw.write("\n");
System.out.println("写入完成");
} catch (IOException e) {
throw new RuntimeException(e);
}
}
public static void main(String[] args) {
String path = "./Experiment06/";
File file = new File(path + "高级程序设计.txt");
if (!file.exists()) {
System.out.println("文件不存在, 正在创建...");
try {
if (file.createNewFile()) {
System.out.println("文件创建成功");
} else {
System.out.println("文件创建失败");
}
} catch (Exception e) {
e.printStackTrace();
}
}
addNumbers(file);
}
}
文件不存在时,先创建再写入:
文件存在时,直接写入:
Answer02
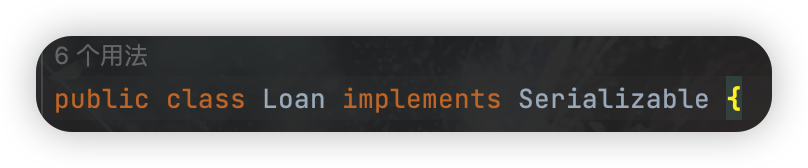
import java.io.*;
/**
* @author Owem
* @date 2022/10/25 16:01
* @description TODO
**/
public class StorageLoanFile {
public static void main(String[] args) {
Loan[] loanList = new Loan[5];
for (int i = 0; i < 5; i++) {
loanList[i] = new Loan("L" + i, Math.random() * 1000);
}
String path = "./Experiment06/";
try (ObjectOutputStream output = new ObjectOutputStream(new FileOutputStream(path + "LoanInfo.dat"))) {
output.writeObject(loanList);
System.out.println("写入完成");
} catch (IOException e) {
throw new RuntimeException(e);
}
}
}
Answer03
import java.io.FileInputStream;
import java.io.ObjectInputStream;
/**
* @author Owem
* @date 2022/10/25 16:16
* @description TODO
**/
public class RecoveryLoanFile {
public static void main(String[] args) {
String path = "./Experiment06/";
double sum = 0;
try (ObjectInputStream input = new ObjectInputStream(new FileInputStream(path + "LoanInfo.dat"))) {
while (true) {
Loan[] newLoans = (Loan[]) (input.readObject());
for (Loan newLoan : newLoans) {
System.out.println(newLoan.toString());
sum += newLoan.getDeposit();
}
}
} catch (Exception ex) {
System.out.println("完成, 总金额: " + sum);
}
}
}
Answer04
import java.io.*;
import java.util.Scanner;
/**
* @author Owem
* @date 2022/10/25 16:21
* @description TODO
**/
public class CountCharacterFrequency {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
int[] frequency = new int[128];
System.out.print("Enter an ASCII file: ");
String path = "./Experiment06/";
File file = new File(path + input.nextLine());
if (!file.exists()) {
System.out.println("文件不存在,正在创建ascii文件");
try (ObjectOutputStream outputStream = new ObjectOutputStream(new BufferedOutputStream(new FileOutputStream(file)))) {
for (int i = 0; i < 1024; i++) {
outputStream.writeUTF((char) ((int) (Math.random() * 128)) + "");
}
} catch (IOException e) {
throw new RuntimeException(e);
}
}
try (ObjectInputStream inputStream = new ObjectInputStream(new BufferedInputStream(new FileInputStream(file)))) {
try {
while (true) {
frequency[inputStream.readUTF().charAt(0)]++;
}
} catch (Exception ignored) {
}
} catch (IOException e) {
throw new RuntimeException(e);
}
for (int i = 0; i < frequency.length; i++) {
if (i == 10) {
System.out.print("character:换行符" + " freq: " + frequency[i] + "\t");
} else {
System.out.print("character:" + i + " freq: " + frequency[i] + "\t\t");
}
if ((i + 1) % 10 == 0) {
System.out.println();
}
}
}
}
本文是原创文章,采用 CC BY-NC-ND 4.0 协议,完整转载请注明来自 Owen
评论
匿名评论
隐私政策
你无需删除空行,直接评论以获取最佳展示效果